ColorsNew
Nord Design System uses a color system to guide our users, create a branded look-and-feel, draw attention to important information, and make content more accessible for everyone.
When designing digital products you can use Nord’s color system to pick color combinations that work harmoniously, adapt based on usage, and provide support for different appearance modes like light and dark.
Principles #
Use sparingly and intentionally #
Nord uses functional coloring that supports products designed to be run on workstations. Color is used to communicate not to decorate. We use color sparingly and intentionally in order to emphasize important information. The color system facilitates all-day use while minimizing visual fatigue.
Utilize color roles #
We offer a curated palette of colors that are intended for specific roles within Nord. For example, we apply consistent coloring to components such as text, status, buttons, and navigation. This helps users to identify a component and understand its relationship to its environment.
Make it accessible #
When the color system is applied, it will be in compliance with color accessibility standards. We support WCAG 2.1 level AA which requires 4.5:1 contrast for text. We never rely solely on color for communication in Nord. Accessibility ensures that our product works for people with visual impairments and improves legibility for all users.
Applying colors to interface #
Nord uses common patterns of color application to create consistency and convey meaning. The below sections explain in detail how to use and apply Nord Design System’s colors in different contexts.
Surface colors #
Surface colors are the default UI surfaces for applications. Surface colors are used for pages, modals, tables, headers, and cards. Surfaces live on top of backgrounds. All content such as text, buttons, forms, and icons should be placed on a surface.
The basic structure of an application in Nord is Background > Surface > Content
.
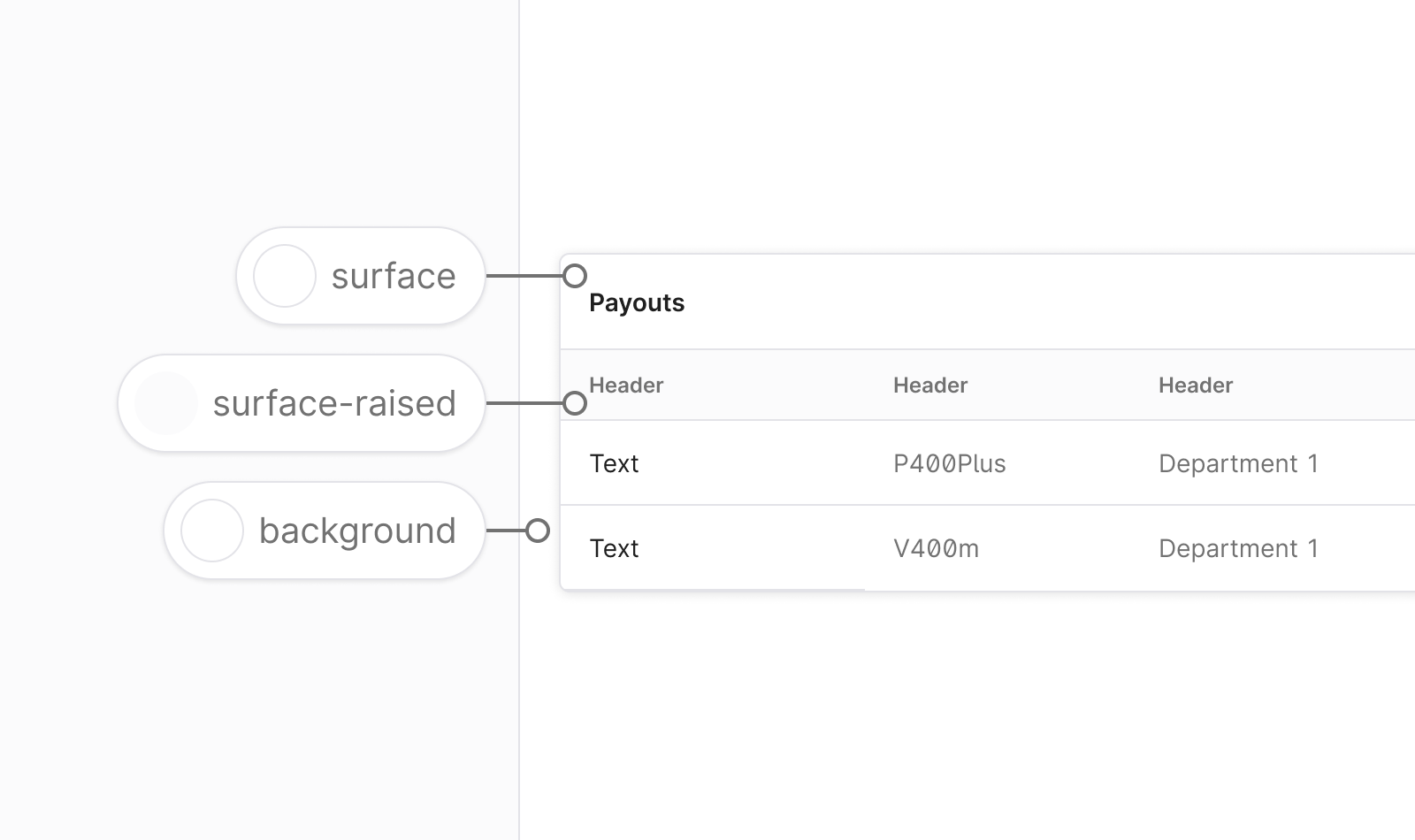
Text colors #
Text always appears on a surface and contains modifiers to indicate hierarchy, status, and importance.
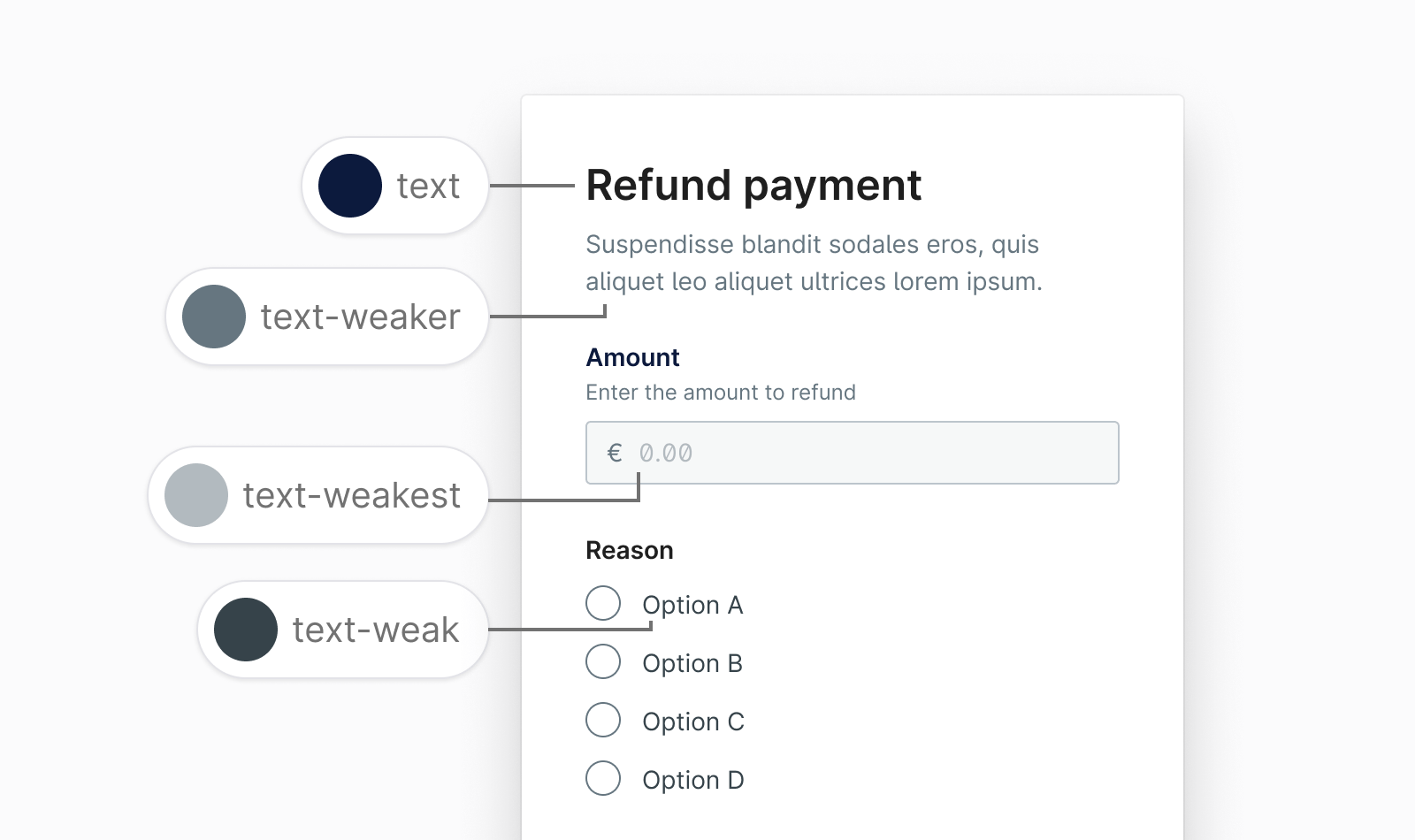
Accent colors #
This is the accent color for a design. It is used as primary button background, hero area background, link color, and selected state.
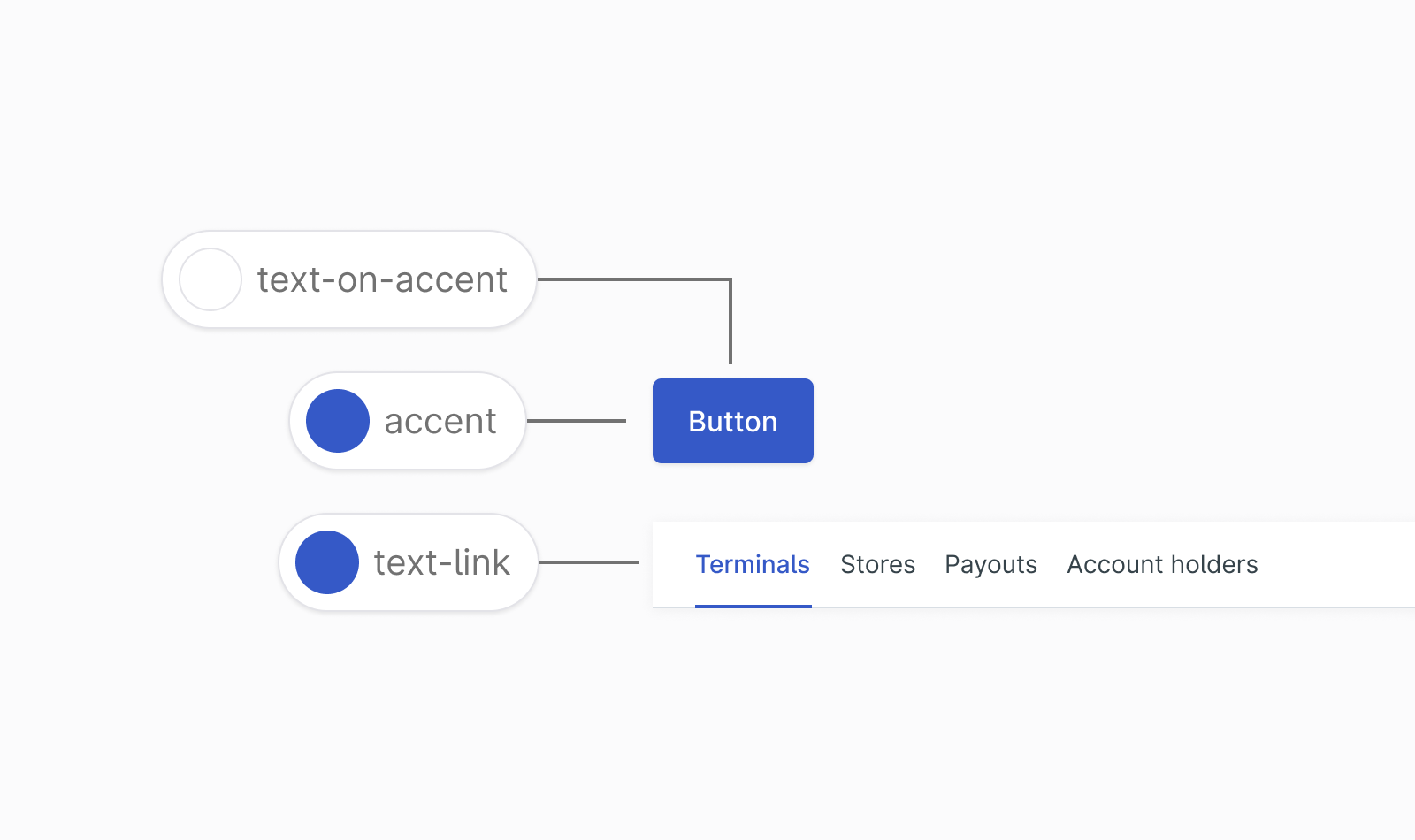
Navigation colors #
This is the color for sidebar navigation. The sidebar color helps to distinguish navigation from other surfaces.
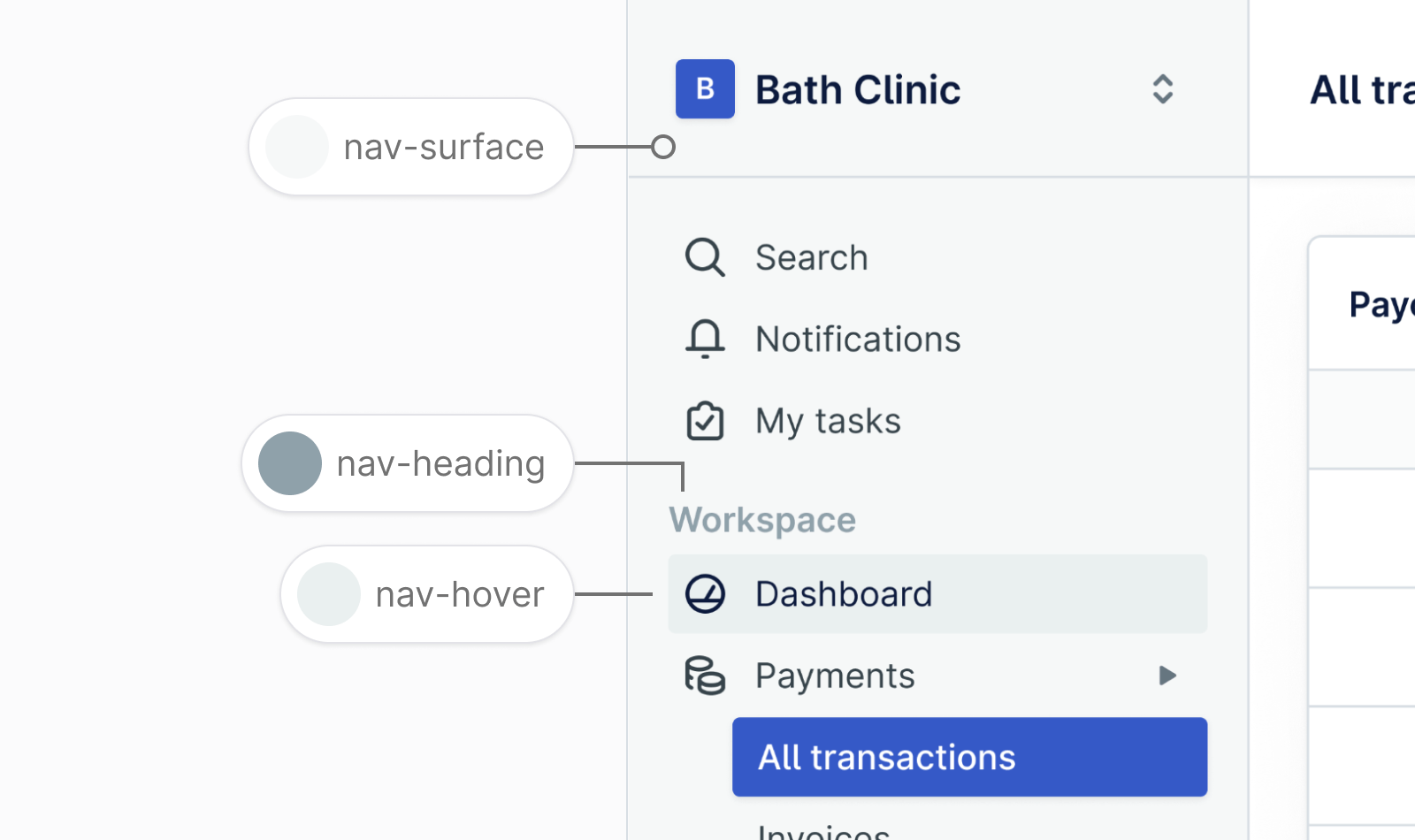
Border colors #
Borders can define the edges of a component or separate content areas.
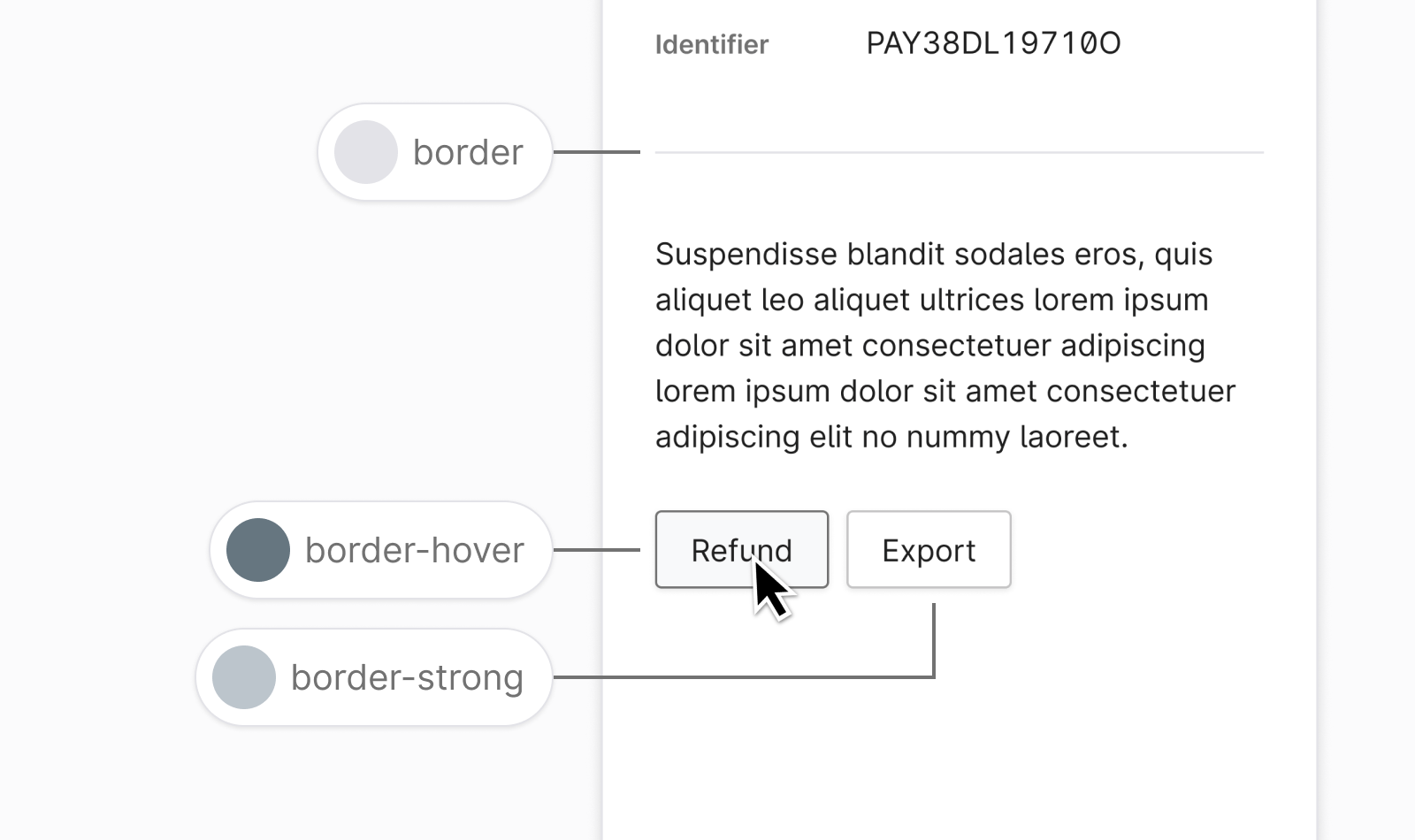
Status colors #
Color can be used to highlight important information by giving it a status. For example the green success status indicates a strong positive state.
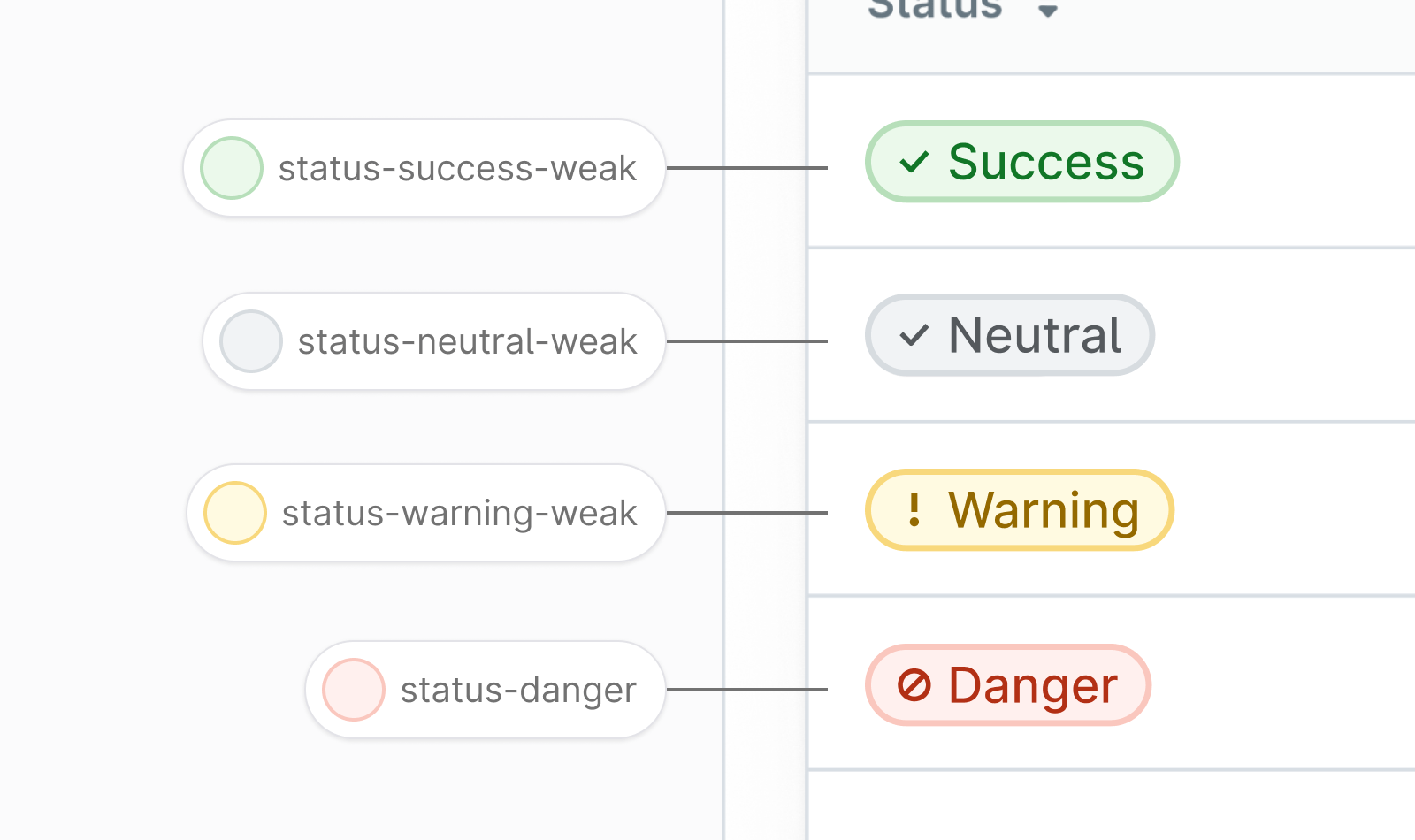
Button colors #
Button colors are used to define the default button style and states in Nord.
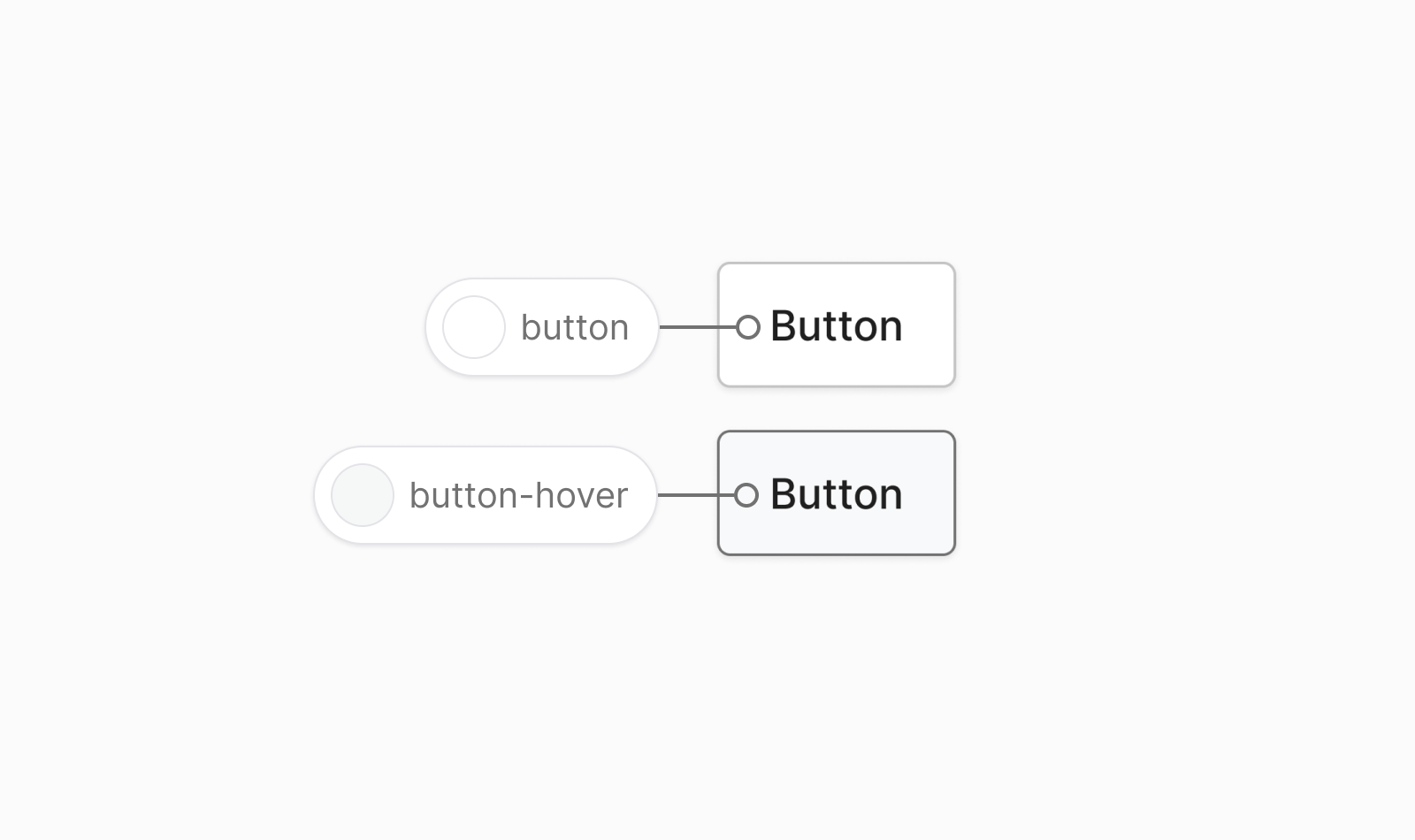
Icon colors #
Icon colors should be used when an icon is placed on a surface. If an icon appears on the accent color, use the text-on-accent color in place of the default icon color.
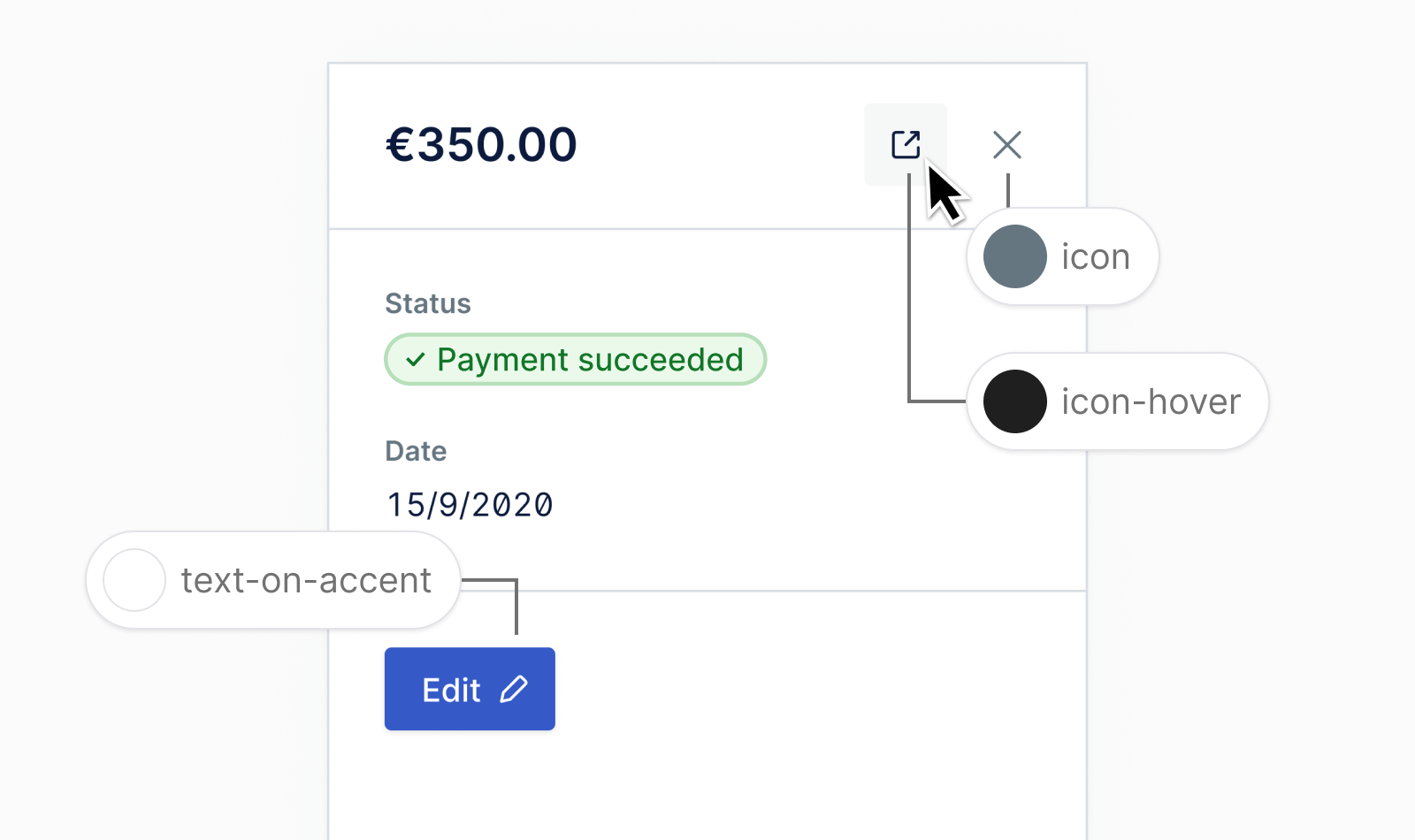
Figma usage #
You can find the color styles from Nord Light Theme and Nord Dark Theme files.
Color utilities #
In addition to the Figma themes, we ship a collection of color utilities in a package called @nordhealth/color
. These are meant for creating and maintaining consistent and accessible color palettes.
Integrating @nordhealth/color
into your project is straightforward. You can start using our color utilities immediately by adding this tag to the <head>
of your application:
<script src="https://nordcdn.net/ds/color/2.0.12/index.min.js" integrity="sha384-jxrTtdfF/6hvgvhLE4alDoHJFk9JrEs860SBDqh8N/S4EMQ6Q9UA3CTRLbAEGY3i" crossorigin="anonymous"></script>
With the above script tag added, you now have access to all of Nord’s color utilities via NordColor
function:
<script>
const textColor = NordColor.getTextColor(backgroundColor)
const contrastRatio = NordColor.getContrastRatio(textColor, backgroundColor)
const colorPalette = NordColor.getColorPalette({ hues: 18 })
const hexToRgb = NordColor.hexToRgb("#3559c7")
const rgbToHex = NordColor.rgbToHex("rgb(53, 89, 199)")
</script>
Installing color utilities #
Before moving further with the installation, please make sure you have Node.js installed on your machine. You can install the latest version through their website.
If you’re planning on using Nord’s Color Utilities in a project that doesn’t yet use Node Package Manager, you’ll have to first create a package.json file. To do this, run npm init
and follow the steps provided. Once finished, you can install Nord’s Color Utilities by following the instructions below.
Run in your project or website root directory (where package.json lives):
# With NPM
npm install @nordhealth/color --save
# With Yarn
yarn add @nordhealth/color
Once installed, you can import the necessary tools from the color package:
// Import one utility
import { getTextColor } from "@nordhealth/color"
// Import all utilities
import { getTextColor, getColorPalette, getContrastRatio, hexToRgb, rgbToHex } from "@nordhealth/color"
Text Color utility #
getTextColor
utility calculates and returns an accessible text color for a chosen background color. The returned text colors are pulled from Nord Design Tokens. Please note that the utility expects the input color to be in HEX
or RGB
format:
// Get accessible text color for the chosen background color
const backgroundColor = "#fafafa"
const textColor = getTextColor(backgroundColor)
This utility can be be used to generate text colors for automatically generated statuses and user generated content. We’re utilizing it in Nord Color Generator and Nord Status Color Generator in addition to Nord Theme Builder to generate the text colors.
Contrast Ratio utility #
getContrastRatio
utility calculates and returns the contrast ratio between two CSS colors. Most commonly used for calculating the contrast between text and background. Please note that the utility expects the input color to be in HEX
or RGB
format:
// Get contrast ratio between two colors
const textColor = "#fafafa"
const backgroundColor = "#fafafa"
const contrastRatio = getContrastRatio(textColor, backgroundColor)
This utility can be be used to determine whether a certain color combination passes the color contrast requirements (4.5:1 or higher contrast). We’re utilizing it in our Design Tokens documentation to determine accessible color combinations.
Color Palette utility #
getColorPalette
utility takes a valid CSS color as the base which is used for generating complementary colors. This utility is meant for creating and maintaining consistent and accessible color palettes. We use it internally to develop our existing Figma color palettes and to test new color variations:
// Generate a color palette with 18 hues
const colorPalette = getColorPalette({ hues: 18 })
This utility can be be used to for example generate status colors programmatically. Combined with getTextColor
utility you can make sure that generated color combinations stay accessible.
getColorPalette
also allows you to set the number of color hues, number of shades, the amount of lightness, and whether gray is included or not:
// Generate a color palette with 12 hues and 1 shade
const colorPalette = getColorPalette({ hues: 12, shades: 1 })
// Generate a color palette with 12 hues, 1 shade and a gray hue
const colorPalette = getColorPalette({ hues: 12, shades: 1, gray: true })
// Generate a color palette with 12 hues and 1 shade, based on a custom accent color
const colorPalette = getColorPalette({ hues: 12, shades: 1, accent: "#2a469d" })
// Generate a color palette with 12 hues and 1 shade, with custom lightness
const colorPalette = getColorPalette({ hues: 12, shades: 1, lightness: 0.7 })
Here’s a list of all available options with their default values:
getColorPalette({
accent: nColorAccent, // {String} Valid CSS color to be used as the base for calculations
hues: 12, // {Number} How many hues should be generated based on the accent color
shades: 1, // {Number} How many shades of each hue should be generated
lightness: 0.5, // {Number} Controls the overall lightness of color palettes (0-1)
gray: false // {Boolean} Whether or not to include a specific gray palette
})
Hex to RGB utility #
hexToRgb
utility converts a HEX color to a RGB color:
// Convert hex to rgb
const hex = "#3559c7"
const rgb = hexToRgb(hex) // outputs "rgb(53, 89, 199)"
RGB to HEX utility #
rgbToHex
utility converts a RGB color to a HEX color:
// Convert hex to rgb
const rgb = "rgb(53, 89, 199)"
const hex = rgbToHex(rgb) // outputs "#3559c7"
For more examples, see Nord Color Generator and Nord Status Color Generator.
Color generator #
Nord Color Generator is a tool that generates color palettes programmatically for use with Nord Themes. While this tool demonstrates the capabilities of the color utilities found from @nordhealth/color
package, it also acts as an internal tool for our team. We use it to develop our existing color palettes and test new color variations.
Getting support #
Have a question about color usage? Please head over to the Support page for more guidelines and ways to contact us.